Python Multithreading
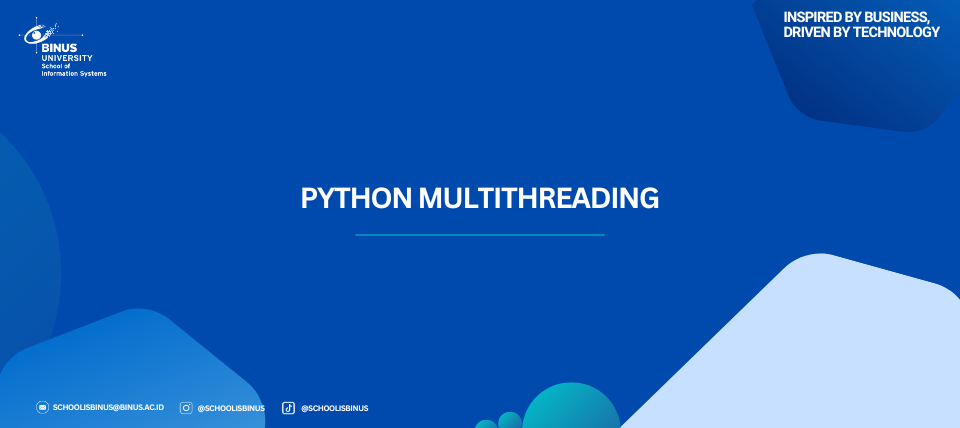
Python Multithreading is a method in Python to run multiple threads in a process simultaneously. With multithreading, we can create the illusion that multiple tasks are being performed in parallel, although in Python, this is often limited by the Global Interpreter Lock (GIL).
Concepts in Python multithreading :
- Thread
A thread is the smallest unit of execution in a program. In Python, threads are lightweight subprocesses that run inside a single main process. All threads share the same memory as the main process.
- Global Interpreter Lock (GIL)
Python uses GIL, a locking mechanism that prevents multiple threads from executing Python bytecode simultaneously. As a result, multithreading does not allow parallel execution for tasks that are CPU-bound (tasks that require a lot of CPU processing). However, multithreading is still effective for I/O-bound tasks (e.g. reading files or accessing the network).
- Threading Module
Python provides a built-in module called threading for multithreading implementation.
Advantages
Responsiveness: Multithreading makes programs more responsive, especially in GUI applications.
Lightweight: Threads are lighter than processes in multiprocessing.
Memory Sharing: All threads share the same memory, making it easy to share data.
Disadvantages
Limited by GIL: Multithreading is not ideal for CPU-bound tasks as GIL limits only one Python thread that can run at a time.
Thread Safety: When multiple threads access shared data, race conditions can occur. You need to use a locking mechanism like Lock to ensure the data is safe.
Debugging multithreading can be difficult due to the concurrent nature of execution